In the age of GitHub CoPilot, have Roslyn Analysers had their day?
Roslyn Analysers have saved my code in the past, identifying issues that I had missed. Now they don't, they have gone backwards. Can GitHub CoPilot fill that gap? The answer is an emphatic YES.
If you're wondering what Roslyn Analysers are, the Microsoft Documentation says
.NET compiler platform (Roslyn) analyzers inspect your C# or Visual Basic code for style, quality, maintainability, design, and other issues. This inspection or analysis happens during design time in all open files.
In the past, when I was a full time developer they had often saved me from problematic code by underlying smelly code before it was checked-in to source-control. I wrote a service years ago which relied on System.Timers where some hadn't been Disposed correctly which could've led to a memory leak. Doing a quick search, this blog from 2011 (yes, 13 years earlier than this post) mentions exactly what I used the analysers for.
Because I'm no longer a full time developer, I don't generally look at them much, but today we had a Microsoft Hackathon, so I thought I'd have a quick play to see what has changed (and improved). To my suprise, the newer Microsoft Analysers didn't find memory leaks or SQL Injection. The older ones are no longer maintained and marked as obsolete.
Here is the code which I was using.
public void MemoryLeak()
{
var list = new List<string>();
for (int i = 0; i < 1000000; i++)
{
list.Add(i.ToString());
}
}
and this
public void SqlInjection(string fromTheInterweb)
{
var sql = "SELECT * FROM Users WHERE Name = '" + fromTheInterweb + "'";
var list = new List<string>();
using (var conn = new SqlConnection("Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;"))
{
conn.Open();
using (var cmd = new SqlCommand(sql, conn))
{
using (var reader = cmd.ExecuteReader())
{
while (reader.Read())
{
list.Add(reader.GetString(0));
}
}
}
}
}
The first bit of code uses a List which is a Disposable type (so if it's not disposed correctly it will cause a memory leak). In the second bit of code there's concatenated strings to build a SQL Command, which could lead to a SQL Injection attack.
When running code analysis, neither of these issues were pointed out. All I got were some fairly generic warnings:
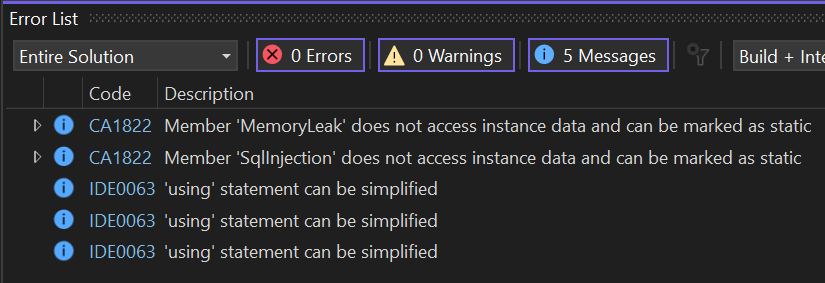
This was disappointing. In my view, Roslyn Analysers have gone backwards.
On the flipside, I also use GitHub CoPilot, which I've previously written about and think it's pretty awesome. I asked CoPilot

and it's response was exactly what I was looking for from the Roslyn Analysers, and it was far more comprehensive.
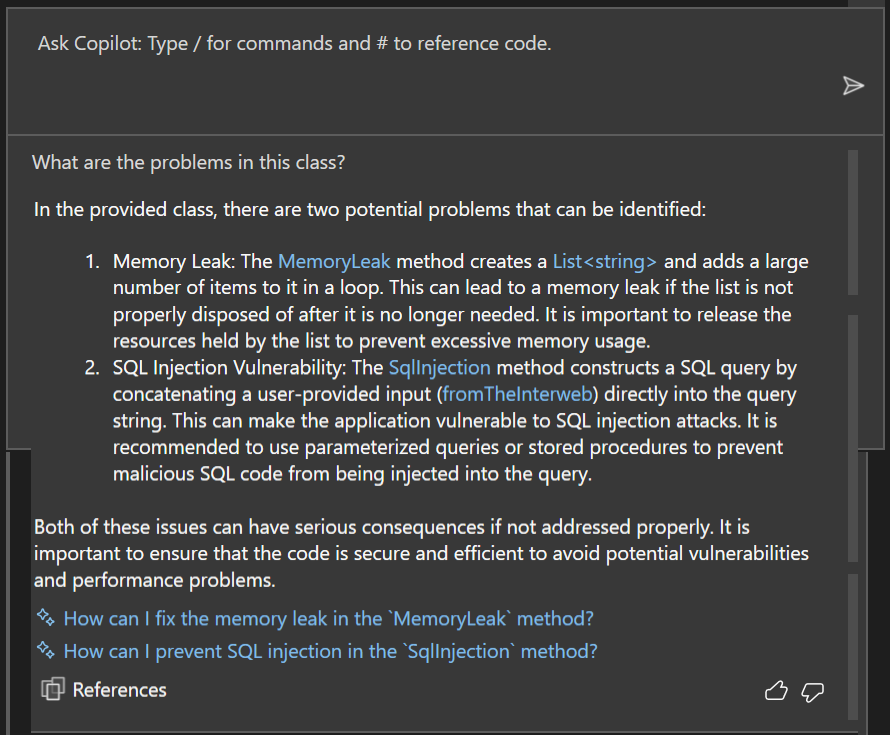
There's still a place for Roslyn Analysers, especially when using 3rd party tools such as SonarLint, Code Cracker and others, but I think moving forward I will be relying on GitHub CoPilot to help me find smelly code.
What are your thoughts? Feel free to leave comments.