Discards in C#
Discards is a useful but obscure new feature of C#. It has some great uses, but also needs a little caution.
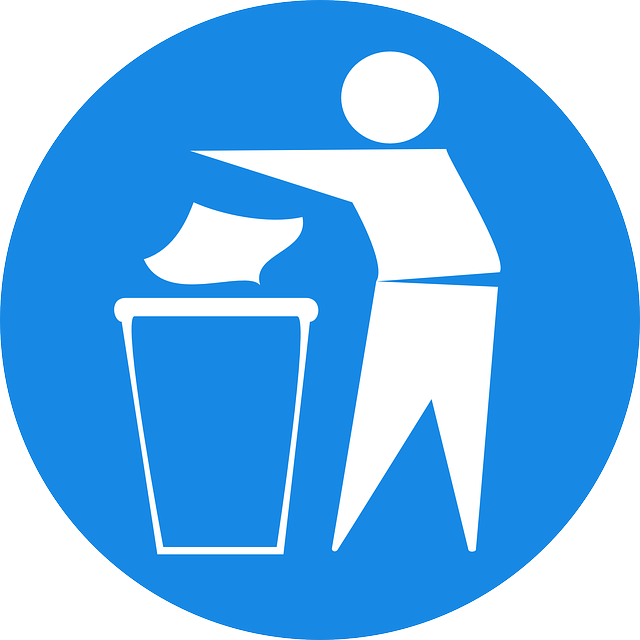
When you discard something, you throw it away. Why would you want to do this in C#?
Firstly, if you're capturing a return value, for example a ValueTuple but are only interested in capturing a small piece of it then a discard is needed to save on copying uneccessary data.
Secondly, C#8 has changed the way switch statements can be written, making the default case a discard, which makes for neater code. I have written another post about that here but read on to undersatnd a bitmore about discards.
Lets see an example:
internal static void DemonstrateDiscards()
{
var simpleValueTuple = (FirstName: "Dominic", LastName: "Batstone", Age: 500);
//capture the retuirned tuple, and the age (int) in a new variable.
//"discard" the two string values because we're not interested in them
var (age,_,_) = Discards(simpleValueTuple);
Console.WriteLine(age);
}
private static (int,string,string) Discards((string FirstName, string LastName, int Age) simpleValueTuple)
{
//SWAP the values, so the age is first
return (simpleValueTuple.Age, simpleValueTuple.FirstName, simpleValueTuple.LastName);
}
In the code snippet, we send a ValueTuple to the method Discards, which returns a Tuple<int,string,string>.
When we capture the return value, we want to assign the int value into the age variable,but discard the two string values.
The Discards are shown by the use of the underscore symbol '_'.
Underscore Confusion
There is a case where using the '_' character can be confusing and possibly lead to unexpected consequences. Have a look at this code;
internal static void UnderscoreConfusion(string _)
{
var message = "This is some text";
_ = message;
Console.WriteLine(_); //result= "This is some text"
}
You'll see the use of '_' is a valid variable name. Therefore instead of being a Discard we can actually assign a value to it. Persoanlly, I think this is confusing and can lead to unexpected behaviour that would be hard to debug. Hopefully this kind of thing coul dbe picked up in a code review, but personally I'd prefer it if '_' was only used for Discards.
There are a coupel of more obscure exampels in the Microsoft Documentation.
Remember to see Discard use in the blog post Switch statement for C# has changed.
This is part of my personal refresh of C# series of blog posts.